Handling form submissions in WordPress can be challenging, especially when dealing with security, performance, and user experience. Thankfully, WordPress provides two built-in mechanisms for processing forms: admin-post.php and admin-ajax.php. Understanding when and how to use them can help you create efficient and secure form submissions.
Understanding Admin-Post and Admin-Ajax
Both admin-post.php and admin-ajax.php offer ways to process form data, but they serve slightly different purposes:
- admin-post.php is generally used for handling form submissions that reload the page or redirect after processing.
- admin-ajax.php is designed for handling submissions via Ajax, which allows updating the page content without reloading.
The choice between them depends on your use case—whether you need a full-page reload or a seamless Ajax submission.
Handling Forms with Admin-Post
admin-post.php works well when submitting forms without JavaScript, typically where you need a full-page redirect after processing. Here’s how you can handle a form submission using this method:
1. Creating the Form
First, create an HTML form in your template or a shortcode:
<form action="<?php echo admin_url('admin-post.php'); ?>" method="post">
<input type="text" name="username" placeholder="Enter your name" required>
<input type="hidden" name="action" value="process_form">
<input type="submit" value="Submit">
</form>
2. Handling the Submission
Next, in your theme’s functions.php or a custom plugin:
function handle_form_submission() {
if (isset($_POST['username'])) {
$username = sanitize_text_field($_POST['username']);
// Process Data (e.g., Store in Database, Send Email, etc.)
wp_redirect(home_url('/thank-you'));
exit;
}
}
add_action('admin_post_process_form', 'handle_form_submission');
add_action('admin_post_nopriv_process_form', 'handle_form_submission');
The two hooks ensure both logged-in and non-logged-in users can submit the form.
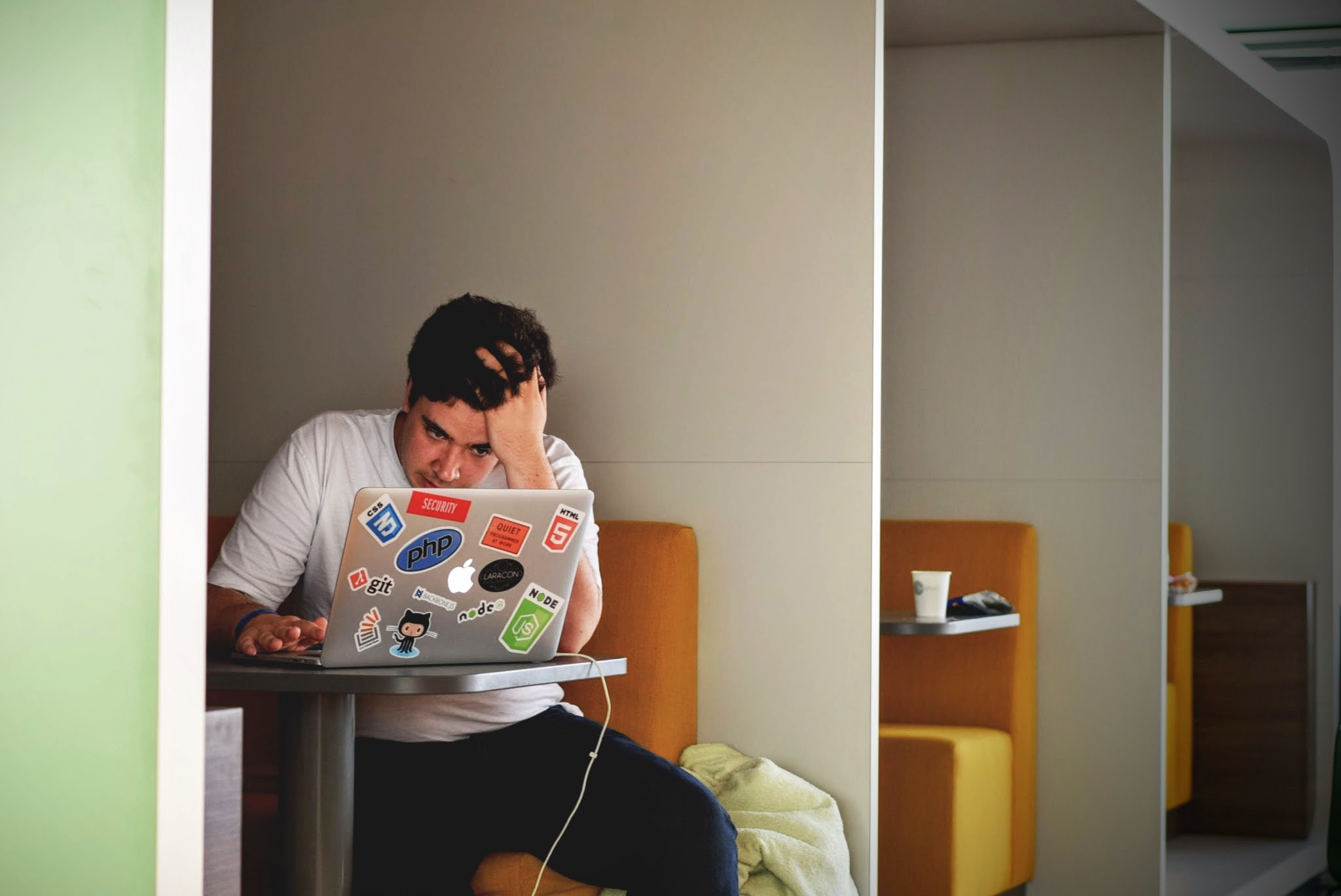
Handling Forms with Admin-Ajax
admin-ajax.php is useful when handling Ajax-based form submissions, which prevent a page reload and enhance the user experience.
1. Creating the Ajax Form
Modify your form to include a custom JavaScript function:
<form id="ajax-form" method="post">
<input type="text" id="username" name="username" placeholder="Enter your name" required>
<button type="button" id="submit-btn">Submit</button>
</form>
2. Adding the JavaScript for Ajax Submission
Include the following JavaScript in your theme or a custom JS file:
jQuery(document).ready(function($){
$('#submit-btn').on('click', function(){
var username = $('#username').val();
$.ajax({
type: 'POST',
url: ajaxurl, // WordPress provides this global variable
data: {
action: 'process_ajax_form',
username: username
},
success: function(response){
alert(response);
}
});
});
});
3. Handling the Ajax Request
Now, write the PHP function to process the Ajax submission:
function handle_ajax_form_submission() {
if (isset($_POST['username'])) {
$username = sanitize_text_field($_POST['username']);
echo "Hello, " . esc_html($username) . "!";
}
wp_die(); // Always use wp_die() to terminate Ajax requests
}
add_action('wp_ajax_process_ajax_form', 'handle_ajax_form_submission');
add_action('wp_ajax_nopriv_process_ajax_form', 'handle_ajax_form_submission');
Now, when users submit the form, it will process without refreshing the page.
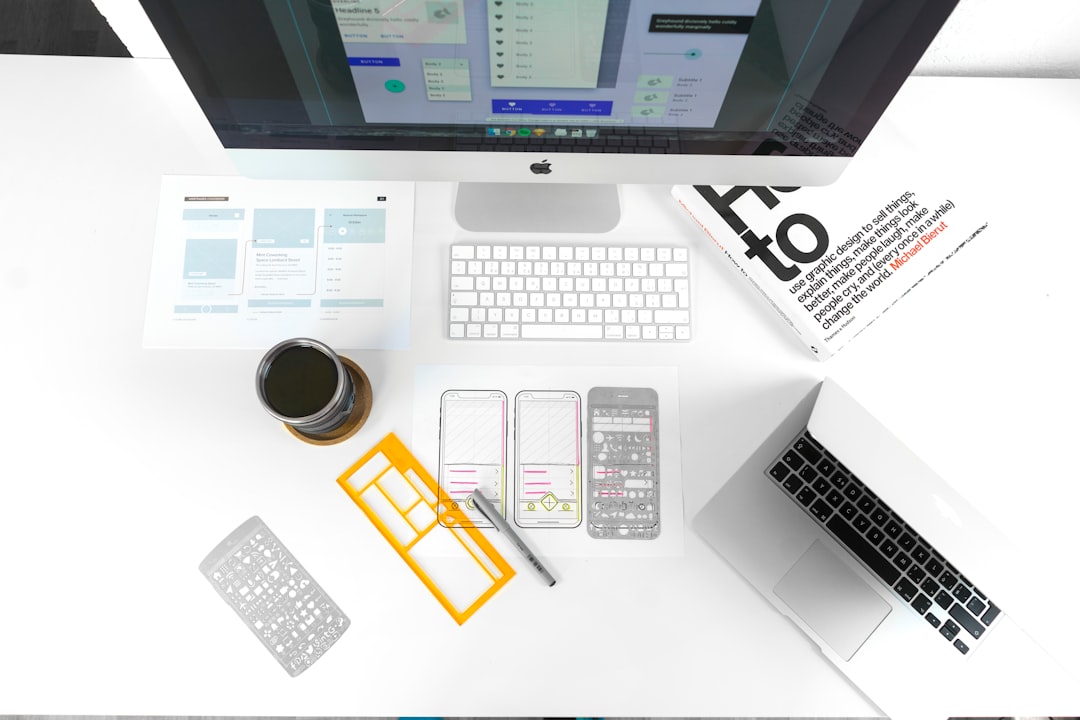
When to Use Each Method?
Choosing between these methods boils down to the user experience you want to provide:
- Use admin-post.php if form submission requires redirection, like logging in, subscribing, or handling payments.
- Use admin-ajax.php if you want a smoother experience, such as live search results, contact forms, or adding items to a cart.
Security Considerations
When handling form submissions, always consider security:
- Use sanitize_text_field() and similar functions to prevent malicious input.
- Always check user permissions when handling sensitive data.
- For Ajax requests, verify requests with nonces to prevent unauthorized submissions.
Conclusion
Both admin-post.php and admin-ajax.php are robust ways to handle forms in WordPress. While admin-post.php is suitable for traditional form submissions with redirections, admin-ajax.php allows for more dynamic interactions with JavaScript. Understanding when to use each method will help you create efficient and user-friendly WordPress forms.