Shortcodes are a powerful feature in WordPress that allow users to easily insert dynamic content into posts, pages, widgets, and even theme files without writing complex code. The do_shortcode()
function is a handy way to execute shortcodes directly within PHP files. Whether you’re a developer or a WordPress enthusiast, understanding how to use do_shortcode()
can greatly improve your site’s functionality.
What is do_shortcode()
and Why Use It?
Normally, shortcodes are added to posts and pages within the WordPress block editor using square brackets. For instance:
However, if you need to use the same shortcode inside a theme file, template, or widget where shortcodes may not be processed automatically, you use do_shortcode()
. This function processes the shortcode and returns the expected output.
How to Use do_shortcode()
in WordPress
The syntax for the function is straightforward:
<?php echo do_shortcode('[your_shortcode]'); ?>
Let’s break it down step by step with a few examples.
1. Using do_shortcode()
in Theme Files
If you are working on a theme and want to display a shortcode within a PHP template file, use the following code:
<?php echo do_shortcode('[contact-form-7 id="123" title="Contact form 1"]'); ?>
This will render the Contact Form 7 shortcode directly inside your theme file.
2. Using do_shortcode()
in Widgets
WordPress does not process shortcodes inside text widgets by default. However, you can enable this by adding the following code to your functions.php file:
add_filter('widget_text', 'do_shortcode');
After adding this snippet, you can now use shortcodes inside text widgets.
3. Using do_shortcode()
with Custom Shortcodes
If you have created a custom shortcode, you can execute it within your theme files:
// Define a simple shortcode function
function my_custom_shortcode() {
return '<p>This is a custom shortcode output!</p>';
}
// Register the shortcode
add_shortcode('my_shortcode', 'my_custom_shortcode');
Now, call this shortcode inside a PHP template file:
<?php echo do_shortcode('[my_shortcode]'); ?>
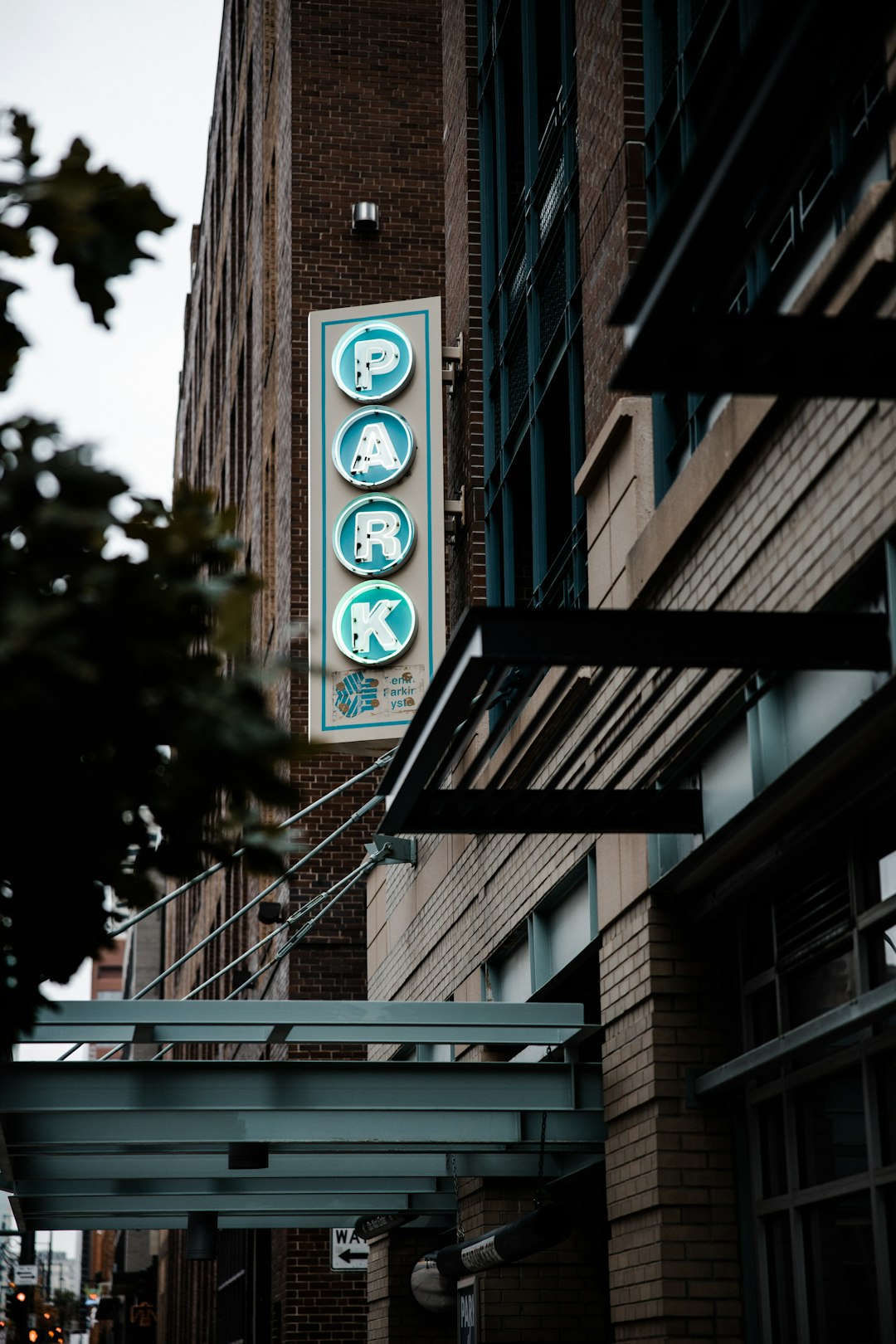
Common Use Cases for do_shortcode()
Using do_shortcode()
is helpful in a variety of scenarios:
- Inside Theme Files: Display dynamic content in templates.
- In Widgets: Enable shortcode functionality in text widgets.
- In Custom Plugin Development: Integrate shortcodes into custom plugins.
- Conditionally Display Content: Use shortcodes within
if
conditions.
Precautions While Using do_shortcode()
Although do_shortcode()
is powerful, there are a few precautions you should take:
- Performance Considerations: Shortcodes that retrieve large amounts of data can slow down page loads.
- Security Risks: Be cautious when accepting user inputs through shortcodes.
- Ensure Shortcode Exists: Before running
do_shortcode()
, check if the shortcode is registered to avoid errors.
[pai-img]performance, security, wordpress[/ai-img]
Alternatives to do_shortcode()
Although do_shortcode()
is useful, sometimes there are better alternatives:
- Directly Calling a Function: If the shortcode is custom, consider calling the function instead.
- Shortcode in Widgets Without Code: Some builder plugins support shortcodes without needing
do_shortcode()
.
Final Thoughts
The do_shortcode()
function is a great tool when integrating shortcodes within WordPress templates, but it should be used wisely. Always ensure it is necessary before implementing it in a theme or plugin to maintain performance and security.