In WordPress development, shortcodes are an essential feature used to insert dynamic content into posts, pages, and widgets effortlessly. However, sometimes shortcodes need to be used directly within PHP files rather than inside the WordPress editor. In such cases, developers can utilize functions to echo a shortcode dynamically. This article explores how to use a function to echo a shortcode in WordPress effectively.
Understanding Shortcodes in WordPress
Shortcodes in WordPress allow users to add complex features without writing extensive code. They are enclosed within square brackets and can be placed in posts, pages, or widgets. For example, a shortcode to display a gallery might look like this:
When WordPress processes the content, it replaces the shortcode with the corresponding output based on the defined functionality.
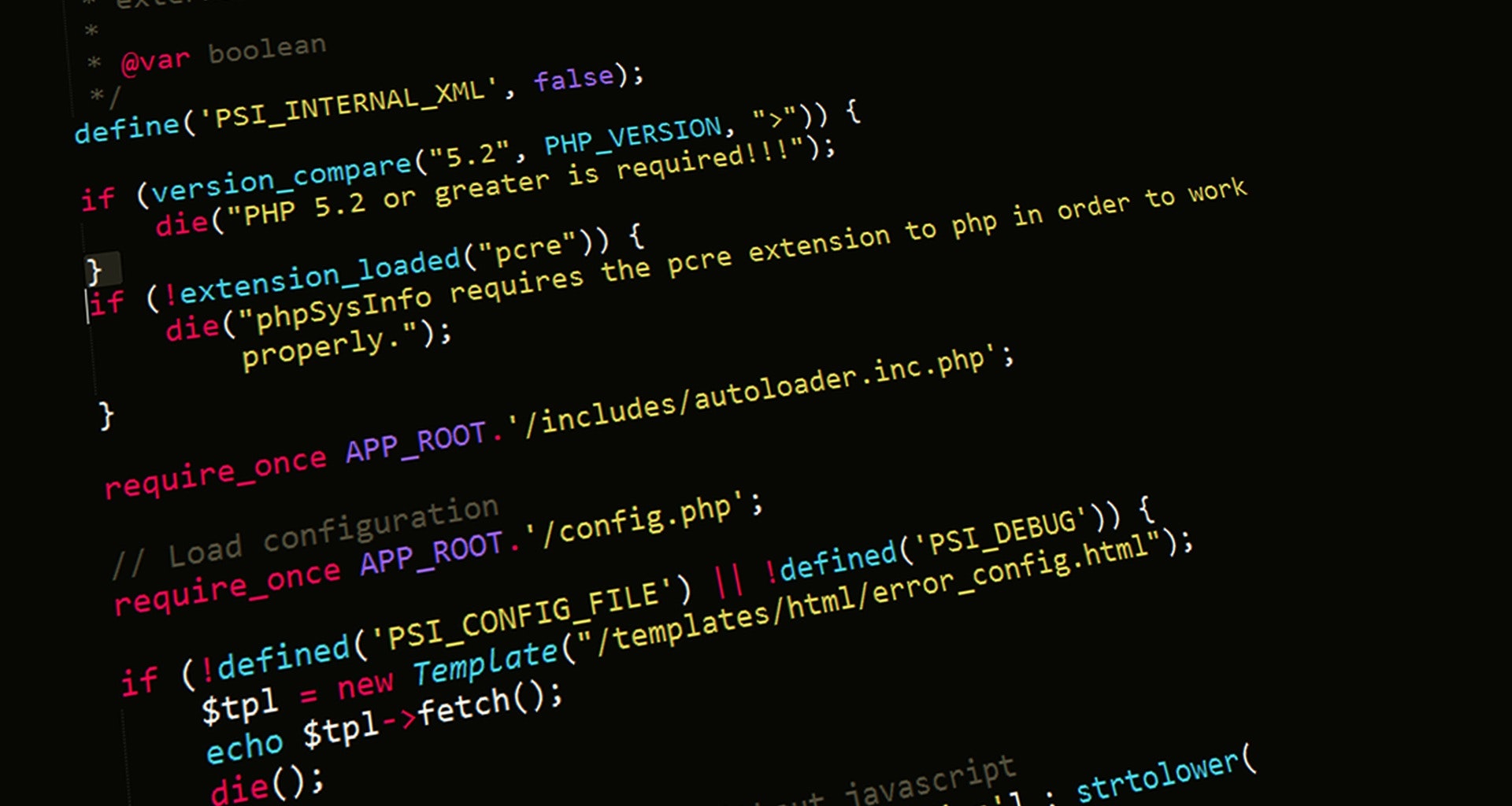
Using PHP to Echo a Shortcode
WordPress provides the do_shortcode()
function, which allows developers to execute shortcodes within PHP files. The general syntax of this function is:
<?php echo do_shortcode('[your_shortcode]'); ?>
By calling do_shortcode()
, WordPress processes the specified shortcode and returns its output as a string.
Example: Embedding a Contact Form via Shortcode
Suppose a website uses the Contact Form 7 plugin, which provides a shortcode like this:
[contact-form-7 id="456" title="Contact form"]
To add this contact form inside a PHP template file, the developer should use:
<?php echo do_shortcode('[contact-form-7 id="456" title="Contact form"]'); ?>
This method ensures that the form appears in the desired location within the theme template.
Creating a Custom Function to Echo a Shortcode
In many cases, wrapping the do_shortcode()
function inside a custom function can help with reusability and maintainability. A simple custom function might look like this:
function display_custom_shortcode() {
echo do_shortcode('[your_shortcode]');
}
The function can then be called anywhere within a PHP template file like so:
<?php display_custom_shortcode(); ?>
Using a dedicated function improves code structure and makes debugging more manageable.
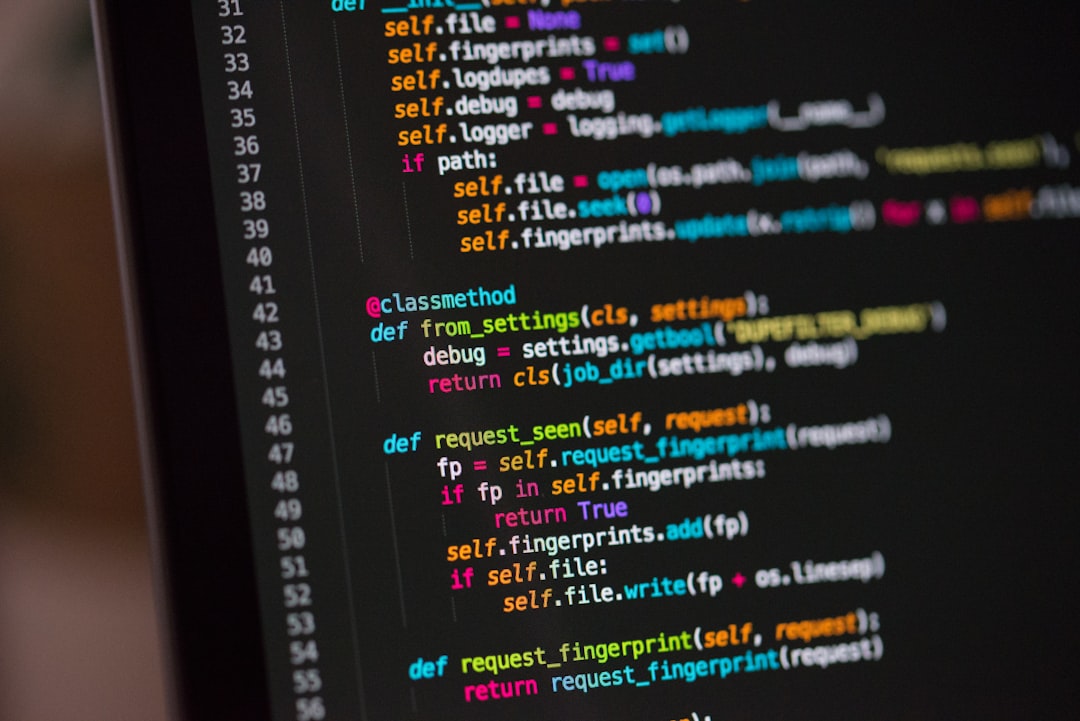
Using Shortcodes Inside Widgets
WordPress does not enable shortcodes in widgets by default. However, adding the following code snippet to the theme’s functions.php
file allows shortcodes to function inside widget areas:
add_filter('widget_text', 'do_shortcode');
This modification ensures that any widgets containing shortcodes will properly display their content instead of showing the raw shortcode text.
Common Use Cases for Echoing Shortcodes
Developers and designers often need to use shortcodes dynamically within theme files. Some popular scenarios include:
- Embedding forms in custom templates
- Displaying sliders in specific sections
- Inserting advertisements dynamically
- Embedding WooCommerce products
By utilizing do_shortcode()
within functions, developers can improve flexibility and control over how shortcodes are displayed.
Conclusion
Using a function to echo a shortcode in WordPress is a powerful technique that provides flexibility in theme and plugin development. Whether embedding a form, a slider, or custom content, developers can leverage do_shortcode()
to dynamically insert shortcodes into PHP templates. Organizing the code with a reusable function also enhances readability and maintainability.
FAQ
1. Can shortcodes be used inside WordPress widgets?
Yes, but by default, shortcodes do not work within widget areas. Adding the add_filter('widget_text', 'do_shortcode');
function to the theme’s functions.php
file enables shortcode execution inside text widgets.
2. Why is my echoed shortcode not displaying correctly?
If a shortcode does not render properly, ensure that the shortcode exists and is registered correctly. Also, verify that do_shortcode()
is being used properly within the PHP file.
3. Can multiple shortcodes be echoed inside a function?
Yes, multiple shortcodes can be combined within a single function using concatenation, like this:
function display_multiple_shortcodes() {
echo do_shortcode('[first_shortcode]');
echo do_shortcode('[second_shortcode]');
}
4. Is it recommended to modify the core WordPress files to add shortcode functionality?
No, modifying core files is discouraged as updates may overwrite customizations. Instead, custom shortcodes should be added via functions in a child theme or a custom plugin.
5. Can shortcodes be used in WordPress themes and plugins?
Yes, shortcodes can be used both in themes and plugins. Plugins often register custom shortcodes, which themes can then implement using do_shortcode()
.