“`html
Pathfinding is a crucial aspect of many applications, ranging from robotics to video game development. One of the most efficient and widely used algorithms for pathfinding is the A* (A-Star) algorithm. In this article, we will explore how to implement A* pathfinding in Java, ensuring that it performs efficiently and correctly.
Understanding the A* Algorithm
The A* algorithm is an informed search algorithm that finds the shortest path between two points on a given graph. It balances performance and accuracy by using a heuristic function to estimate the cost from the current node to the target. The algorithm follows these key steps:
- Start from the initial node (source).
- Evaluate its neighboring nodes.
- Use the function
f(n) = g(n) + h(n)
, where:g(n)
is the cost from the start node ton
.h(n)
is the estimated cost fromn
to the goal.
- Always expand the node with the lowest
f(n)
value. - Repeat until the goal is reached.
By continually selecting nodes that minimize the estimated total cost, A* finds an optimal path efficiently.
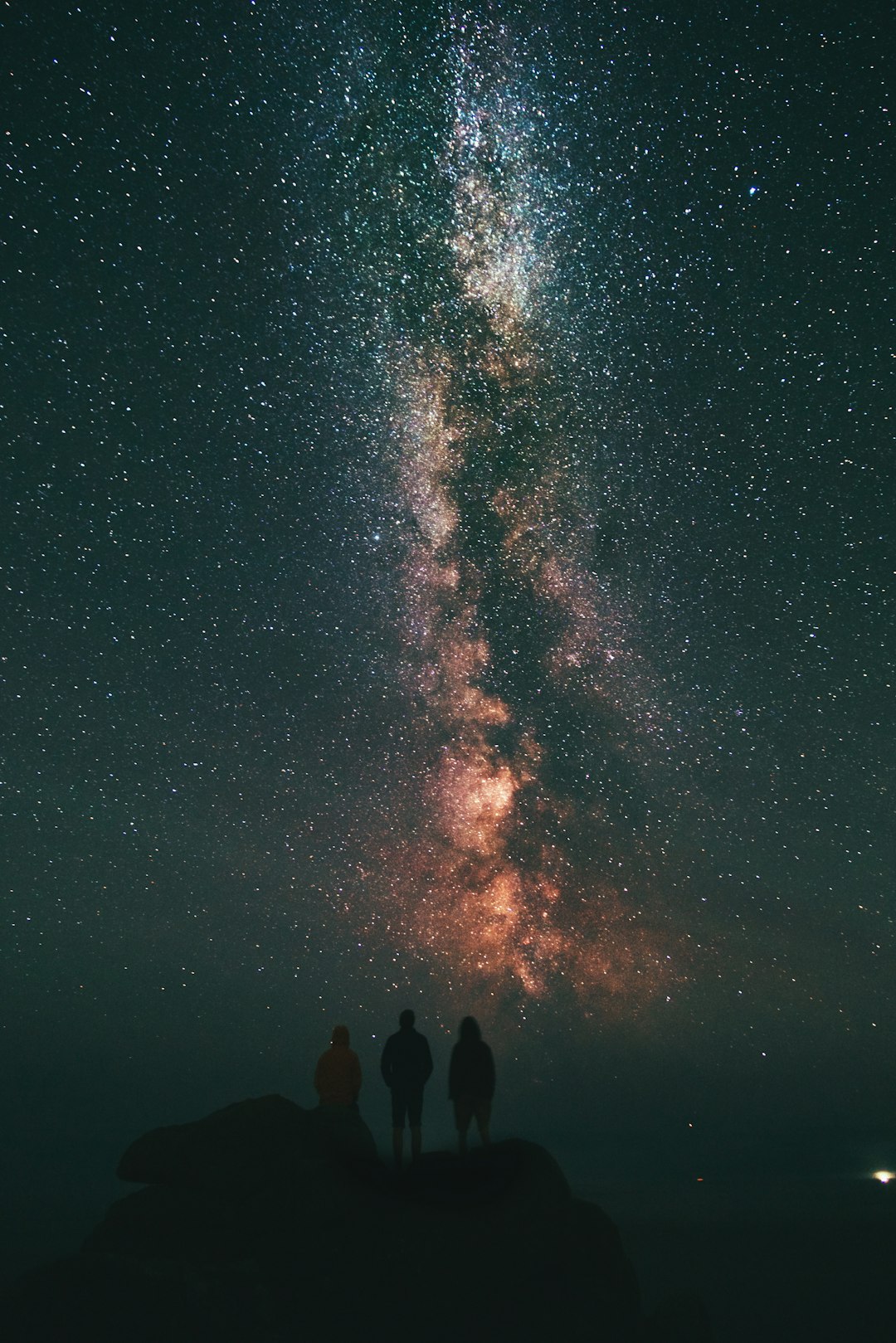
Implementing A* in Java
Now, let’s walk through a simple Java implementation of the A* algorithm. The implementation consists of the following components:
- A Node class to represent points in the grid.
- A Priority Queue to always explore the most promising node first.
- A Grid Representation to model the environment.
The Node Class
Each node in the grid must store its position, cost values, and a reference to its parent for path reconstruction.
class Node implements Comparable {
int x, y;
int gCost, hCost;
Node parent;
public Node(int x, int y) {
this.x = x;
this.y = y;
}
public int getFCost() {
return gCost + hCost;
}
@Override
public int compareTo(Node other) {
return Integer.compare(this.getFCost(), other.getFCost());
}
}
The compareTo
method allows us to store nodes in a priority queue, ensuring that the most promising one is expanded first.
Implementing the A* Algorithm
Now, let’s write the actual A* function:
import java.util.*;
public class AStarPathfinding {
private static final int[][] DIRECTIONS = {{0,1}, {1,0}, {0,-1}, {-1,0}};
public static List findPath(Node start, Node goal, int[][] grid) {
PriorityQueue<Node> openSet = new PriorityQueue<>();
Set<Node> closedSet = new HashSet<>();
openSet.add(start);
while (!openSet.isEmpty()) {
Node current = openSet.poll();
if (current.x == goal.x && current.y == goal.y) {
return reconstructPath(current);
}
closedSet.add(current);
for (int[] dir : DIRECTIONS) {
int newX = current.x + dir[0];
int newY = current.y + dir[1];
if (!isValid(newX, newY, grid) || closedSet.contains(new Node(newX, newY))) {
continue;
}
Node neighbor = new Node(newX, newY);
neighbor.gCost = current.gCost + 1;
neighbor.hCost = heuristic(neighbor, goal);
neighbor.parent = current;
if (!openSet.contains(neighbor)) {
openSet.add(neighbor);
}
}
}
return Collections.emptyList();
}
private static List reconstructPath(Node node) {
List path = new ArrayList();
while (node != null) {
path.add(node);
node = node.parent;
}
Collections.reverse(path);
return path;
}
private static int heuristic(Node a, Node b) {
return Math.abs(a.x - b.x) + Math.abs(a.y - b.y); // Manhattan Distance
}
private static boolean isValid(int x, int y, int[][] grid) {
return x >= 0 && y >= 0 && x < grid.length && y < grid[0].length && grid[x][y] == 0;
}
}
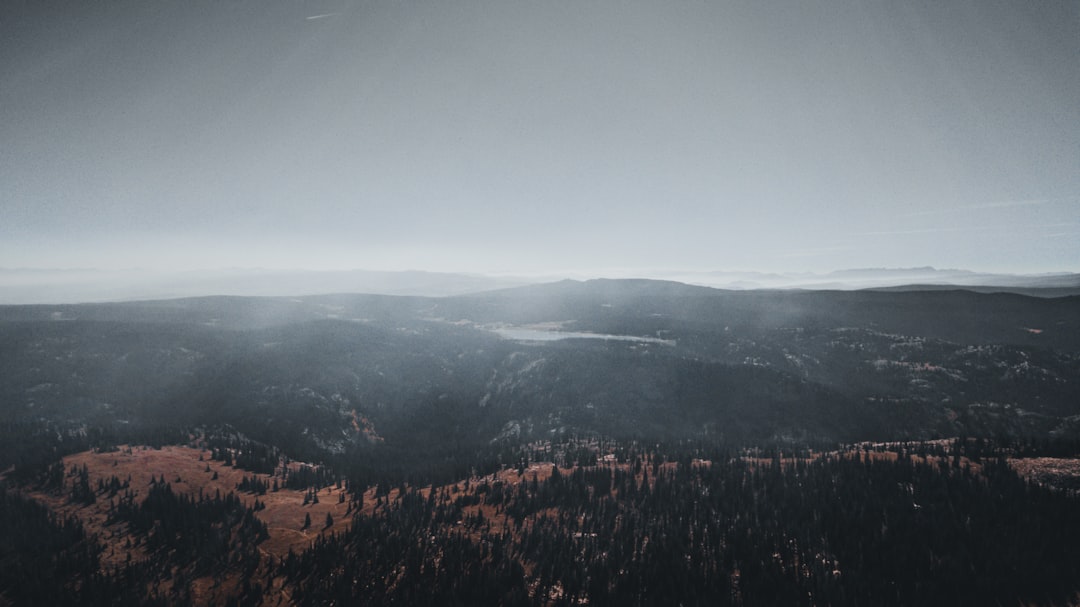
Optimizing the Algorithm
To further optimize A*, consider the following improvements:
- Use a binary heap or Fibonacci heap for better priority queue performance.
- Implement early exit conditions to avoid unnecessary computations.
- Use weighted heuristics to adjust performance based on specific needs.
Conclusion
The A* pathfinding algorithm is one of the most effective ways to find the shortest route in a given environment. By leveraging both the actual cost and estimated cost, it ensures an optimal path with high efficiency.
With a proper implementation in Java, A* can be applied to numerous applications, from robotics to video game AI. Optimizing the algorithm further can significantly improve performance, making it suitable for large-scale projects.
“`